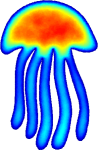 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
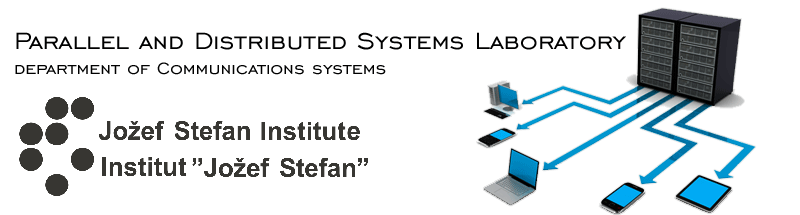 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_APPROXIMATIONS_RBFINTERPOLANT_FWD_HPP_
2 #define MEDUSA_BITS_APPROXIMATIONS_RBFINTERPOLANT_FWD_HPP_
33 template <
typename RBFType,
typename vec_t>
65 const Eigen::Matrix<scalar_t, Eigen::Dynamic, 1>&
coefficients);
72 template <
typename operator_t>
86 #endif // MEDUSA_BITS_APPROXIMATIONS_RBFINTERPOLANT_FWD_HPP_
Root namespace for the whole library.
Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > coefficients_
Coefficients (for scaled fn.)
Scalar scalar_t
Type of the elements, alias of Scalar.
vector_t point_
Center point.
rbf_t rbf_
RBF used for interpolation.
scalar_t scale() const
Get the scale.
const vector_t & point() const
Get the center point.
const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > & coefficients() const
Get the coefficient vector.
A class representing Monomial basis.
Class representing a RBF Interpolant over a set of nodes of the form.
RBFType rbf_t
Radial basis function type.
vec_t::scalar_t scalar_t
Scalar type.
Monomials< vec_t > mon_
Augmenting monomials.
vec_t vector_t
Vector type.
RBFInterpolant(const rbf_t &rbf, const Monomials< vec_t > &mon, const vector_t &point, const std::vector< vector_t > &support, scalar_t scale, const Eigen::Matrix< scalar_t, Eigen::Dynamic, 1 > &coefficients)
Construct a RBF interpolant with known coefficients.
scalar_t operator()(const vector_t &point) const
Evaluate the interpolant at given point.
std::vector< vector_t > support_
Local scaled stencil points.