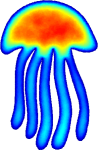 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
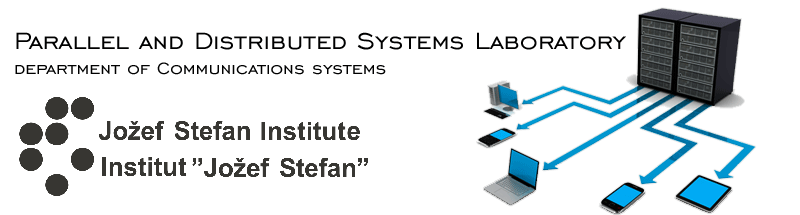 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_APPROXIMATIONS_OPERATORS_FWD_HPP_
2 #define MEDUSA_BITS_APPROXIMATIONS_OPERATORS_FWD_HPP_
32 template <
typename Derived>
35 static constexpr
int size() {
return 1; }
36 static constexpr
int index(Derived) {
return 0; }
37 std::string name()
const {
return Derived::name(); }
38 static std::string type_name() {
return Derived::type_name(); }
44 std::array<Derived, 1>
operators()
const {
return {*
static_cast<const Derived*
>(
this)}; }
63 template <
typename basis_t>
65 const basis_t& basis,
int index,
typename basis_t::vector_t point,
66 const std::vector<typename basis_t::vector_t>& support,
73 template <
typename basis_t>
75 const basis_t& basis,
int index,
76 const std::vector<typename basis_t::vector_t>& support,
80 template <
typename Derived>
84 template <
int dimension>
85 struct Lap :
public Operator<Lap<dimension>> {
87 enum {
dim = dimension };
88 static std::string name() {
return format(
"Laplacian<%d>",
dim); }
89 static std::string type_name() {
return name(); }
96 template <
int dimension>
99 enum {
dim = dimension };
101 static int size() {
return dim; }
102 static std::string name() {
return format(
"Der1s<%d>",
dim); }
103 static std::string type_name() {
return name(); }
106 static std::array<Der1<dimension>, dimension>
operators();
119 template <
int dimension>
124 static int size() {
return dim * (
dim + 1) / 2; }
125 static std::string name() {
return format(
"Der2s<%d>",
dim); }
126 static std::string type_name() {
return name(); }
129 static std::array<Der2<dimension>, dimension * (dimension + 1) / 2>
operators();
140 template <
int dimension>
141 struct Der1 :
public Operator<Der1<dimension>> {
156 std::string
name()
const;
163 template <
int dimension>
164 struct Der2 :
public Operator<Der2<dimension>> {
190 std::string
name()
const;
200 template <
int dimension>
201 struct Derivative :
public Operator<Derivative<dimension>> {
210 std::string
name() {
return format(
"Derivative<%d> wrt. %s",
dim,
orders); }
212 static std::string
type_name() {
return format(
"Derivative<%d>",
dim); }
217 #endif // MEDUSA_BITS_APPROXIMATIONS_OPERATORS_FWD_HPP_
Base class for a differential operator.
Root namespace for the whole library.
Scalar scalar_t
Type of the elements, alias of Scalar.
Derivative(const std::array< int, dim > &orders)
Parameter orders represent the derivative orders .
std::array< int, dim > orders
Values .
@ dim
Dimensionality of the domain.
basis_t::scalar_t applyAt0(const basis_t &basis, int index, const std::vector< typename basis_t::vector_t > &support, typename basis_t::scalar_t scale) const
Like apply, but with point equal to 0.
std::ostream & operator<<(std::ostream &os, const Gaussian< S > &b)
Output basic information about given Gaussian RBF.
Der2()
Default constructor for array preallocation purposes.
Der2< dim > operator_t
Type of this family's members.
int var
Index representing derived variable (x = 0, y = 1, z = 2)
static int index(Der1< dim > d)
Get index of operator d in the family.
static std::string type_name()
Human readable type name.
static std::string type_name()
Human readable type name.
@ dim
Dimensionality of the domain.
@ dim
Dimensionality of the domain.
Represents a family of all second derivatives.
int var2
Higher index representing derived variable (x = 0, y = 1, z = 2, ...)
Represents a second derivative wrt. var1 and var2.
static std::string type_name()
Human readable type name.
Der1()
Default constructor for array preallocation purposes.
basis_t::scalar_t apply(const basis_t &basis, int index, typename basis_t::vector_t point, const std::vector< typename basis_t::vector_t > &support, typename basis_t::scalar_t scale) const
Apply this operator to a given basis function.
Der1< dim > operator_t
Type of this family's members.
std::array< Derived, 1 > operators() const
Return an iterable of iterators represented by a family.
Represents a first derivative wrt. var.
static std::array< Der2< dimension >, dimension *(dimension+1)/2 > operators()
Return an iterable of iterators represented by a family.
@ dim
Dimensionality of the domain.
std::string name()
Human readable name.
@ dim
Dimensionality of the domain.
Derived operator_t
Type of the contained operators.
std::string name() const
Human readable name.
Der2(int var)
Initialization of specific 2nd derivative operator.
@ dim
Dimensionality of the domain.
static int index(Der2< dim > d)
Get index of operator d in the family.
Represents a family of all first derivatives.
int var1
Lower index representing derived variable (x = 0, y = 1, z = 2, ...)
std::string name() const
Human readable name.
static std::array< Der1< dimension >, dimension > operators()
Return an iterable of iterators represented by a family.