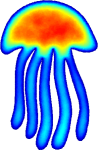 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
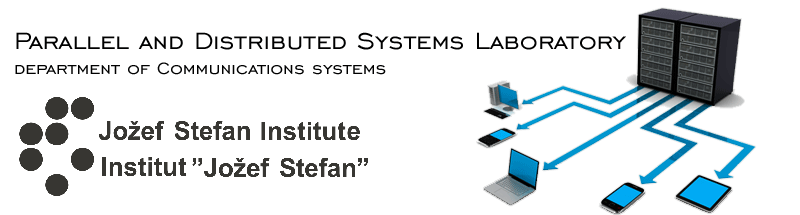 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_IO_HDF_FWD_HPP_
2 #define MEDUSA_BITS_IO_HDF_FWD_HPP_
18 #include <type_traits>
22 template <
typename Derived>
25 template<
typename _Scalar,
int _Rows,
int _Cols,
int _Options,
int _MaxRows,
int _MaxCols>
29 template <
template <
typename...>
class base,
typename derived>
30 struct is_base_of_template_impl {
31 template<
typename... Ts>
32 static constexpr std::true_type test(
const base<Ts...> *);
33 static constexpr std::false_type test(...);
34 using type = decltype(test(std::declval<derived*>()));
37 template <
template <
typename...>
class base,
typename derived>
38 using is_base_of_template =
typename is_base_of_template_impl<base, derived>::type;
91 static std::string
str(
Mode mode);
120 void open(
const std::string&
filename,
const std::string& group_name =
"/",
202 void writeAttribute(
const std::string& attr_name,
const T& value,
const hid_t& type,
203 bool overwrite =
false)
const;
214 template <
typename T>
215 std::pair<std::vector<hsize_t>, std::vector<T>>
228 std::vector<T>
read1DArray(
const std::string& dataset_name)
const;
240 std::vector<std::vector<T>>
read2DArray(
const std::string& dataset_name)
const;
252 std::vector<std::vector<std::vector<T>>>
read3DArray(
const std::string& dataset_name)
const;
264 template<
int dim,
typename T>
266 const std::array<hsize_t, dim>& sizes, hid_t type,
bool overwrite)
const;
279 template<
typename T,
typename array_t>
280 void write1DArray(
const std::string& dataset_name,
const array_t& value,
281 hid_t type,
bool overwrite)
const;
295 template<
typename T,
class array_t>
296 void write2DArray(
const std::string& dataset_name,
const array_t& value, hid_t type,
297 bool overwrite)
const {
298 write2DArray<T>(dataset_name, value, type, overwrite,
299 is_base_of_template<Eigen::MatrixBase, array_t>());
304 template<
typename T,
class array_t>
305 void write2DArray(
const std::string&,
const array_t&, hid_t,
bool, std::false_type)
const;
308 template<
typename T,
class array_t>
309 void write2DArray(
const std::string&,
const array_t&, hid_t,
bool, std::true_type)
const {
310 static_assert(!std::is_same<T, T>::value,
311 "This function produces wrong results for Eigen matrices. Use writeEigen instead.");
327 template<
typename T,
class array_t>
328 void write3DArray(
const std::string& dataset_name,
const array_t& value, hid_t type,
329 bool overwrite)
const;
349 void writeIntAttribute(
const std::string& attr_name,
int value,
bool overwrite =
false)
const;
351 void writeBoolAttribute(
const std::string& attr_name,
bool value,
bool overwrite =
false)
const;
354 bool overwrite =
false)
const;
357 bool overwrite =
false)
const;
364 bool overwrite =
false)
const;
367 std::vector<int>
readIntArray(
const std::string& dataset_name)
const;
369 std::vector<double>
readDoubleArray(
const std::string& dataset_name)
const;
371 std::vector<float>
readFloatArray(
const std::string& dataset_name)
const;
374 template <
typename array_t>
375 void writeIntArray(
const std::string& dataset_name,
const array_t& value,
376 bool overwrite =
false)
const;
378 template <
typename array_t>
379 void writeDoubleArray(
const std::string& dataset_name,
const array_t& value,
380 bool overwrite =
false)
const;
382 template <
typename array_t>
383 void writeFloatArray(
const std::string& dataset_name,
const array_t& value,
384 bool overwrite =
false)
const;
387 std::vector<std::vector<int>>
readInt2DArray(
const std::string& dataset_name)
const;
389 std::vector<std::vector<double>>
readDouble2DArray(
const std::string& dataset_name)
const;
391 std::vector<std::vector<float>>
readFloat2DArray(
const std::string& dataset_name)
const;
394 template <
typename array_t>
395 void writeInt2DArray(
const std::string& dataset_name,
const array_t& value,
396 bool overwrite =
false)
const;
398 template <
typename array_t>
400 bool overwrite =
false)
const;
402 template <
typename array_t>
404 bool overwrite =
false)
const;
408 const std::string& dataset_name)
const;
411 const std::string& dataset_name)
const;
414 const std::string& dataset_name)
const;
417 template <
typename array_t>
418 void writeInt3DArray(
const std::string& dataset_name,
const array_t& value,
419 bool overwrite =
false)
const;
421 template <
typename array_t>
423 bool overwrite =
false)
const;
425 template <
typename array_t>
427 bool overwrite =
false)
const;
437 template <
typename scalar_t =
double>
444 template <
typename Derived>
445 void writeEigen(
const std::string& dataset_name,
const Eigen::MatrixBase<Derived>& value,
446 bool overwrite =
false)
const;
460 template <
typename SparseMatrixType>
461 void writeSparseMatrix(
const std::string& name, SparseMatrixType& matrix,
bool one_based =
true,
462 bool overwrite =
false);
480 template <
typename domain_t>
481 void writeDomain(
const std::string& name,
const domain_t& domain,
bool overwrite =
false);
496 template <
typename timer_t>
497 void writeTimer(
const std::string& name,
const timer_t& timer,
bool overwrite =
false);
509 template <
typename conf_t>
510 void writeXML(
const std::string& name,
const conf_t& conf,
bool overwrite =
false);
530 const H5L_info_t*,
void* data);
562 os <<
"HDF reader for file '" << hdf.
filename()
563 <<
"' with current group '" << hdf.
groupName() <<
"'. ";
564 os <<
"The file is " << (hdf.
isFileOpen() ?
"open" :
"closed")
565 <<
" and the group is " << (hdf.
isGroupOpen() ?
"open" :
"closed") <<
'.';
572 #endif // MEDUSA_BITS_IO_HDF_FWD_HPP_
std::vector< int > readIntArray(const std::string &dataset_name) const
Read given dataset into vector of ints.
void write2DArray(const std::string &dataset_name, const array_t &value, hid_t type, bool overwrite) const
Writes given 2D array of given value and type to file.
Root namespace for the whole library.
static std::string str(Mode mode)
Convert HDF::Mode enum to string.
Scalar scalar_t
Type of the elements, alias of Scalar.
hid_t getGroupID() const
Return raw HDF C group identifier.
std::vector< std::vector< std::vector< double > > > readDouble3DArray(const std::string &dataset_name) const
Read given dataset into 3D vector of doubles.
void writeFloatArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 1D array of floatss.
void reopenGroup()
Reopens current group.
float readFloatAttribute(const std::string &attr_name) const
Reads float attribute.
std::vector< double > readDoubleArray(const std::string &dataset_name) const
Read given dataset into vector of doubles.
void close() const
Closes all open objects. Alias of closeFile().
void write1DArray(const std::string &dataset_name, const array_t &value, hid_t type, bool overwrite) const
Writes given 1D array with given value and type to file.
std::vector< std::string > datasets
Names of datasets.
static herr_t memberIterateCallback(hid_t loc_id, const char *name, const H5L_info_t *, void *data)
Callback required by HDF Literate function when getting a list of members.
std::vector< std::vector< std::vector< float > > > readFloat3DArray(const std::string &dataset_name) const
Read given dataset into 3D vector of floats.
std::vector< std::string > unknowns
Names of unknown objects.
void openFile(const std::string &filename, Mode mode=APPEND)
Opens a given file with a given mode.
void closeFile() const
Closes current file and all objects associated with it (e.g. the group), if open.
void writeTimer(const std::string &name, const timer_t &timer, bool overwrite=false)
Writes given timer to file.
void reopen(Mode mode=APPEND)
Reopens current file and group.
std::vector< std::vector< float > > readFloat2DArray(const std::string &dataset_name) const
Read given dataset into 2D vector of floats.
std::vector< std::vector< T > > read2DArray(const std::string &dataset_name) const
Read given dataset into 2D array of vectors.
std::vector< std::vector< double > > readDouble2DArray(const std::string &dataset_name) const
Read given dataset into 2D vector of doubles.
void writeDoubleAttribute(const std::string &attr_name, double value, bool overwrite=false) const
Write double attribute.
static hid_t openFileHelper(const std::string &filename, Mode mode)
Opens given file with given mode.
hid_t group
Currently open group identifier.
void setGroupName(const std::string &group_name)
Set new group name without opening the new group. Any previously opened group is closed.
std::pair< std::vector< hsize_t >, std::vector< T > > readLinearArray(const std::string &dataset_name) const
Read given dataset into linear dim-D array.
@ READONLY
Read only open, the file must exist.
Simplified HDF5 I/O utilities.
void writeEigen(const std::string &dataset_name, const Eigen::MatrixBase< Derived > &value, bool overwrite=false) const
Write given Eigen expression as a 2D array of its type (either int, double or float).
bool isFileOpen() const
Returns true is currently specified file is open and false otherwise.
void setFilename(const std::string &filename)
Sets new filename without opening the new file. Any previously opened file is closed.
void writeFloat3DArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 3D array of floats.
std::vector< T > read1DArray(const std::string &dataset_name) const
Read 1D dataset given by dataset_name.
~HDF()
Closes all opened objects, by using close().
std::string group_name_
Current group name.
void writeIntArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 1D array of ints.
void writeStringAttribute(const std::string &attr_name, const std::string &value, bool overwrite=false) const
Write string attribute.
std::vector< std::vector< std::vector< int > > > readInt3DArray(const std::string &dataset_name) const
Read given dataset into 3D vector of ints.
std::vector< std::vector< int > > readInt2DArray(const std::string &dataset_name) const
Read given dataset into 2D vector of ints.
void write3DArray(const std::string &dataset_name, const array_t &value, hid_t type, bool overwrite) const
Writes given 3D array of given value and type to file.
bool readBoolAttribute(const std::string &attr_name) const
Reads bool attribute.
Mode
Possible file opening modes.
std::vector< std::vector< std::vector< T > > > read3DArray(const std::string &dataset_name) const
Read given dataset into 3D array of vectors.
std::vector< std::string > groups
Names of subgroups.
T readAttribute(const std::string &attr_name) const
Read attribute given by attr_name.
hid_t getFileID() const
Return raw HDF C file identifier.
void writeInt2DArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 2D array of ints.
void writeFloat2DArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 2D array of floats.
void open(const std::string &filename, const std::string &group_name="/", Mode mode=APPEND)
Opens given file and group.
void writeInt3DArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 3D array of ints.
friend std::ostream & operator<<(std::ostream &os, const Members &members)
Output this type in a user friendly way for quick inspection.
std::string readStringAttribute(const std::string &attr_name) const
Reads non-null-terminated string attribute.
bool isGroupOpen() const
Returns true is currently specified group is open and false otherwise.
std::string filename_
Current file name.
double readDoubleAttribute(const std::string &attr_name) const
Reads double attribute.
HDF()
Construct an empty HDF reader.
void reopenFile(Mode mode=APPEND)
Reopens the current file.
std::vector< std::string > datatypes
Names of datatypes.
const std::string & groupName() const
Get current group name.
const std::string & filename() const
Get current filename.
Eigen::Matrix< scalar_t, -1, -1, 0, -1, -1 > readEigen(const std::string &dataset_name) const
Reads data as an Eigen matrix of type scalar_t.
Members members() const
Returns an object with categorized names of all members of current group.
void writeIntAttribute(const std::string &attr_name, int value, bool overwrite=false) const
Write int attribute.
void closeGroup() const
Closes current group, if open.
void flush() const
Flush opened file, if it is open.
HDF(std::string filename, Mode mode=APPEND)
Construct a HDF reader and open filename with given mode.
void writeDouble2DArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 2D array of doubles.
@ DESTROY
Removes old contents, if any exist.
int readIntAttribute(const std::string &attr_name) const
Reads int attribute.
void writeLinearArray(const std::string &dataset_name, const T *value, const std::array< hsize_t, dim > &sizes, hid_t type, bool overwrite) const
Writes a dim-dimensional dataset stored in 1D array in col-major fashion.
void writeAttribute(const std::string &attr_name, const T &value, const hid_t &type, bool overwrite=false) const
Writes given attribute with given value and type to file.
Matrix(const Scalar &s)
Construct matrix from scalar. Enabled only for fixed size matrices.
std::vector< std::string > groups() const
Returns names of all subgroups in current group in alphabetical order.
void writeDoubleArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 1D array of doubles.
void write2DArray(const std::string &, const array_t &, hid_t, bool, std::true_type) const
Overload for static_assert for Eigen due to different size method.
std::vector< std::string > datasets() const
Returns names of all datasets in current group in alphabetical order.
void writeDouble3DArray(const std::string &dataset_name, const array_t &value, bool overwrite=false) const
Write given value as a 3D array of doubles.
void writeDomain(const std::string &name, const domain_t &domain, bool overwrite=false)
Writes given domain discretization to file.
void writeFloatAttribute(const std::string &attr_name, float value, bool overwrite=false) const
Write float attribute.
@ APPEND
Appends to the existing contents, if any exist.
void setFileName(const std::string &filename)
Alias of setFilename().
const std::string & fileName() const
Alias of filename().
void openGroup(std::string group_name)
Open given group, closing any previously opened group().
void writeXML(const std::string &name, const conf_t &conf, bool overwrite=false)
Writes given XML object to file.
friend std::ostream & operator<<(std::ostream &os, const HDF &hdf)
Output basic info about HDF reader, such as current group and file.
hid_t file
Currently open file identifier.
void writeSparseMatrix(const std::string &name, SparseMatrixType &matrix, bool one_based=true, bool overwrite=false)
Writes a sparse matrix.
std::string readNullTerminatedStringAttribute(const std::string &attr_name) const
Reads null-terminated string attribute.
std::vector< float > readFloatArray(const std::string &dataset_name) const
Read given dataset into vector of floats.
void writeBoolAttribute(const std::string &attr_name, bool value, bool overwrite=false) const
Write bool attribute.
Holds categorized names for all members of a group.
HDF atomic() const
Allows for "atomic" read and write operations to HDF5 files.