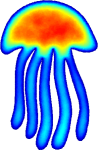 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
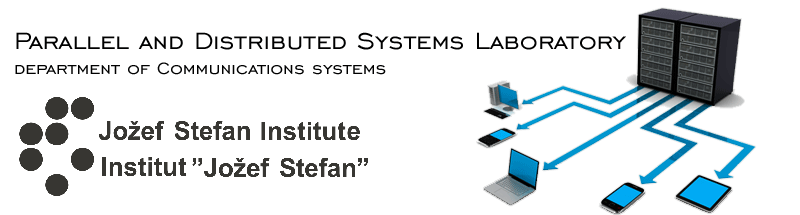 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_DOMAINS_FINDBALANCEDSUPPORT_FWD_HPP_
2 #define MEDUSA_BITS_DOMAINS_FINDBALANCEDSUPPORT_FWD_HPP_
56 template <
typename domain_t>
60 template <
typename domain_t,
typename kdtree_t>
62 domain_t& domain,
const kdtree_t& tree,
int i,
int min_support_size,
63 int max_support_size,
const Range<int>& search_among,
bool force_self);
67 template <
class vec_t>
75 template <
class vec_t>
77 std::vector<bool>& octants_covered,
78 const Eigen::Matrix<typename vec_t::scalar_t, vec_t::dim, -1>& basis);
83 #endif // MEDUSA_BITS_DOMAINS_FINDBALANCEDSUPPORT_FWD_HPP_
static Range< int > balancedSupport(domain_t &domain, const kdtree_t &tree, int i, int min_support_size, int max_support_size, const Range< int > &search_among, bool force_self)
Finds support for a given node i.
Root namespace for the whole library.
int min_support_
Minimal support size.
Scalar scalar_t
Type of the elements, alias of Scalar.
static Eigen::Matrix< typename vec_t::scalar_t, vec_t::dim, vec_t::dim-1 > getFrame(const vec_t &normal)
Gets orthonormal basis of vectors perpendicular to normal.
FindBalancedSupport & forceSelf(bool b=true)
Put each node as the first of its support, even if it is not included in searchAmong().
@ dim
Number of elements of this matrix.
Range< int > search_among_
Search only among these nodes.
void operator()(domain_t &domain) const
Find support for nodes in domain.
static bool mark_quadrant(const vec_t &dx, typename vec_t::scalar_t tol, std::vector< bool > &octants_covered, const Eigen::Matrix< typename vec_t::scalar_t, vec_t::dim, -1 > &basis)
Marks a quadrant in which dx resides as occupied.
FindBalancedSupport & minSupportSize(int size)
Set minimum support size. This overrides the size set in constructor.
int max_support_
Maximal support size.
bool force_self_
Force each node as the first element of its support.
std::vector< int > indexes_t
Class representing a collection of indices.
Class representing the engine for finding directionally balanced supports.
FindBalancedSupport & maxSupportSize(int size)
Set maximal support size. This overrides the size set in constructor.
FindBalancedSupport & searchAmong(indexes_t search_among)
Search only among given nodes.
FindBalancedSupport(int min_support, int max_support)
Constructs an engine with given min and max support sizes.
Matrix(const Scalar &s)
Construct matrix from scalar. Enabled only for fixed size matrices.
FindBalancedSupport & forNodes(indexes_t for_which)
Find support only for these nodes.
Range< int > for_which_
Find support only for these nodes.