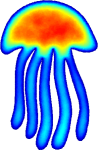 |
Medusa
1.1
Coordinate Free Mehless Method implementation
|
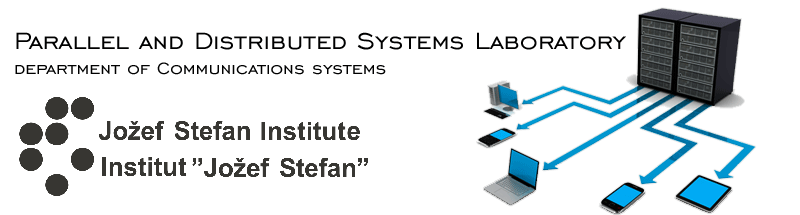 |
Go to the documentation of this file. 1 #ifndef MEDUSA_BITS_UTILS_MEMUTILS_HPP_
2 #define MEDUSA_BITS_UTILS_MEMUTILS_HPP_
30 class deep_copy_unique_ptr :
public std::unique_ptr<T, std::default_delete<T>> {
32 using std::unique_ptr<T, std::default_delete<T>>::unique_ptr;
33 using std::unique_ptr<T, std::default_delete<T>>::operator=;
34 using std::unique_ptr<T, std::default_delete<T>>::reset;
38 std::unique_ptr<T, std::default_delete<T>>(v.clone()) {}
42 std::unique_ptr<T, std::default_delete<T>>() {
43 reset(o ? o->clone() :
nullptr);
48 std::unique_ptr<T, std::default_delete<T>>(std::move(o)) { }
58 reset(o ? o->clone() :
nullptr);
64 std::unique_ptr<T, std::default_delete<T>>::operator=(std::move(o));
74 std::string
mem2str(std::size_t bytes);
82 template<
typename container_t>
84 return sizeof(v[0]) * v.size();
89 #endif // MEDUSA_BITS_UTILS_MEMUTILS_HPP_
deep_copy_unique_ptr(const T &v)
Construct by polymorphically cloning a given value.
Root namespace for the whole library.
deep_copy_unique_ptr & operator=(const deep_copy_unique_ptr &o)
Copy assign by cloning the value of o.
deep_copy_unique_ptr & operator=(const T &v)
Copy assign by cloning a given value.
deep_copy_unique_ptr(const deep_copy_unique_ptr &o)
Copy by cloning the value of o.
deep_copy_unique_ptr(deep_copy_unique_ptr &&o) noexcept
Move construct as unique_ptr.
std::size_t mem_used(const container_t &v)
Returns number of bytes the container uses in memory.
deep_copy_unique_ptr & operator=(deep_copy_unique_ptr &&o) noexcept
Move assign as unique_ptr
Unique pointer with polymorphic deep copy semantics.
std::string mem2str(std::size_t bytes)
Simple function to help format memory amounts for printing.